Introduction to React js
Welcome to our guide on React js, a powerful JavaScript library for building dynamic user interfaces. In this comprehensive tutorial, you will learn how to effectively use React js to create scalable web applications.
What is React js?
React js is a declarative, efficient, and flexible JavaScript library used for building user interfaces. It enables developers to create large web applications that can update and render efficiently with changing data without reloading the page. React js works only on user interfaces in the application, which corresponds to the view in the Model-View-Controller (MVC) template.
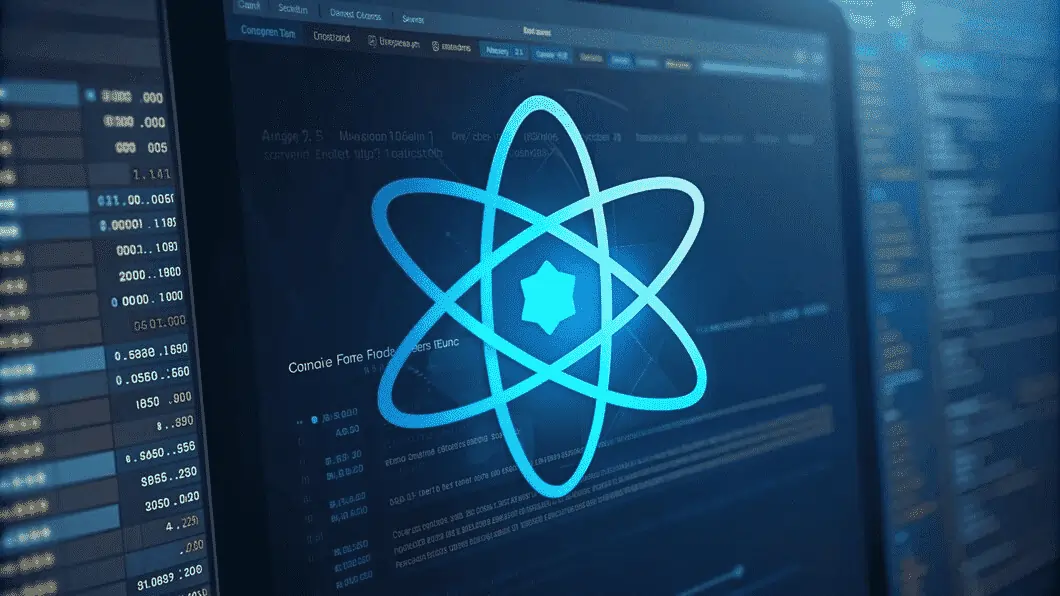
Key Features of React js for Modern Development
Component-Based Architecture
React js applications are built using components, which are reusable pieces of code that manage their own state. Components make your code more modular and easier to maintain.
Virtual DOM
React js uses a virtual DOM to optimize rendering and ensure that the application is highly responsive. This allows for fast updates by applying only the necessary changes to the actual DOM.
JSX – A Syntax Extension
JSX is a syntax extension for JavaScript that allows developers to write HTML-like syntax in their React js components. This makes the code easier to read and write while keeping the logic and structure in one place.
One-Way Data Binding
React js implements one-way data binding, ensuring that changes in the application’s state trigger updates to the user interface. This makes tracking and debugging changes more predictable.
Why Use React?
- Reusable Components: With React, you can create reusable components, saving you time and reducing redundancy in your code.
- High Performance: React’s use of the virtual DOM improves performance by minimizing unnecessary updates to the real DOM.
- Great Ecosystem: React has a vast ecosystem with a variety of tools, libraries, and extensions to enhance the development experience. The community around React is huge, so finding resources and tutorials is easy.
- Scalability: Whether you’re building a small app or a complex web application, React scales well with its flexible architecture.
Integrating React with AI
Integrating AI capabilities into React applications can greatly enhance user experience and functionality. Here’s how you can leverage AI in your React projects:
1. Enhanced User Interactions
Using AI, you can create smarter user interfaces that adapt to user behavior. For example, implementing natural language processing (NLP) can allow your application to understand and respond to user queries in real-time, making interactions feel more intuitive.
2. Predictive Analytics
By incorporating AI-driven analytics into your React app, you can provide users with personalized recommendations based on their behavior and preferences. This is especially useful in e-commerce applications, where AI can analyze user interactions to suggest products they may be interested in.
3. Chatbots and Virtual Assistants
Integrating AI-powered chatbots in your React application can significantly improve customer service. These chatbots can handle common inquiries, provide support, and even guide users through complex processes, all while maintaining a seamless experience.
4. Image and Speech Recognition
React can be combined with AI technologies to implement features such as image recognition and speech-to-text capabilities. This is particularly useful in applications that require enhanced accessibility or content generation.
5. Machine Learning Integration
By leveraging libraries like TensorFlow.js or Brain.js, you can create machine learning models that run directly in the browser. This allows for real-time predictions and processing without the need for server-side computation, enhancing the performance of your React application.
Getting Started with React js: A Beginner’s Guide
To get started with React, you’ll need Node.js installed on your system. After that, you can set up a new React project using the following command:
npx create-react-app my-app
cd my-app
npm start
This will create a new React project and start the development server, allowing you to see your application in action.
Understanding Components in React js
Components in React are like JavaScript functions, but they return HTML via JSX. There are two types of components: functional components and class components.
- Functional Components: These are simple functions that return JSX. They are easier to write and maintain.
- Class Components: Class components have more features like state and lifecycle methods but are now being gradually replaced by functional components with hooks.
Example of a Functional Component:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
State and Props in React
State and props are the core concepts that drive React components. Props are passed from parent to child components, while state is managed within a component.
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
Utilizing Hooks Effectively in React js
With React 16.8, hooks were introduced, allowing functional components to manage state and lifecycle events. Popular hooks include useState
, useEffect
, and useContext
.
import React, { useState } from ‘react’;
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
}
Conclusion
React continues to dominate the world of front-end development with its flexibility, performance, and component-driven architecture. Learning React will equip you with the tools to create dynamic, user-friendly applications that scale well. With React’s vast ecosystem, you can easily integrate other libraries and tools to further extend the functionality of your applications.
Whether you are a beginner or an experienced developer, React offers endless possibilities for building modern web applications. Start your journey with React today and unlock the full potential of front-end development.
Call to Action
Ready to elevate your React development skills? Start implementing these best practices for building dynamic web apps with React in your next project to enhance performance and maintainability. Join the SIW community for more expert insights, tutorials, and resources to keep you updated on the latest trends in web development. Share your thoughts in the comments below—what practices do you find most effective? Let’s learn and grow together!